In this part of the tutorial, we will extend the basic Django DRF API built in the last part to handle creating, updating, and deleting items.
This post is part of the Dockerized Django Back-end API with Angular Front-end Tutorial. Check out all the parts of the tutorial there.
To get to the point where we’ve finished in the last tutorial, use:
$ git checkout v1.2
Implementing the POST Method Using APIView
At the moment, our API is read-only as we can only retrieve Task
objects. In this section, we’ll implement a POST method for creating new tasks.
Instead of using the generic ListAPIView
view, let’s go back to the basic APIView
to see things more explicitly. Modify todoapi/views.py
as below:
Let’s add this import to todoapi/views.py
:
from rest_framework import status
Then, add the POST method to the TaskList
class:
Here, we check that the deserialization was valid before we save the new task to the database. If all goes OK, we send back the created task with a HTTP 201 Created status. Otherwise, we send a 400 Bad Request with the errors from the serializer.
Let’s test the POST method using Postman.
Open Postman and on a new tab, select POST from the drop-down request menu.
Next, enter http://localhost/api/task/ as the request URL. After, click the Body subtab and choose raw. To the right of this, select JSON (application/json) from the drop-down list instead of Text.
Now enter the following JSON body in the text box below:
Click Send. At the bottom of the screen, you should see the returned JSON string:
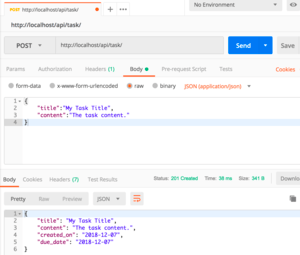
If you now refresh the http://localhost/api/task/ page in your browser, you should see your new task returned with the other dummy data. Alternatively, you can also send a GET request from Postman by choosing GET from the request menu.
You can checkout the code at this stage:
$ git checkout v1.3
Implementing the POST Method Using ListCreateAPIView
Actually, we don’t need to explicitly implement the POST method. The ListCreateAPIView
generic view provides the POST method out-of-the-box. Modify todoapi/views.py
as follows:
The ListCreateAPIView
does all the validation behind the scenes using the specified serializer.
Handling Updates and Deletions
In this section, we will implement handling individual object details. This is needed if we want to delete or update a specific Task
object.
Firstly, we need to modify the TaskSerializer
class to also return the ID of the task. Change todoapi/serializers.py
:
Implementation using APIView
Let’s implement a new TaskDetail
view in todoapi/views.py
. Add the imports:
And the new detail view:
We also need to map the new view to a URL, so edit todoproj/urls.py
:
We are now able to send GET, PUT and DELETE requests to a specific task using a link such as /api/task/1/
. This allows us to retrieve, update or remove an existing task. The task_id
is a URL keyword argument used for object lookup.
Implementation using RetrieveUpdateDestroyAPIView
Finally, let’s use generic views and refactor the TaskDetail
class to be more compact. For this, update todoapi/views.py
using the RetrieveUpdateDestroyAPIView view:
Here, lookup_url_kwarg
specifies which URL keyword argument should be used for object lookup.
In the end, we have the same functionality as the previous more explicit implementation using APIView
.
To get to this point in the tutorial, use:
$ git checkout v1.4
Summary
In this part of the tutorial, we have extended our Django DRF API to handle creation, update and deletion of individual Task
items.
In the next part, we’ll build a dockerized Angular app that will be used to interact with the Django API.
Credit: I liked the approach used in this blog post of first explaining how to implement the HTTP methods manually before using generic views, so I also followed it in this tutorial.