In this blog post, I’ll briefly describe the simple factory pseudopattern.
This post is part of the Design Patterns in Java Series. Check out the full list of covered patterns there.
Why is the Simple Factory a Pseudopattern?
First of all, as I mentioned in the article on the factory method pattern, I use the prefix pseudo because the simple factory isn’t actually considered a pattern in the Gang of Four book.
Nevertheless, I’ve decided to write a blog post on this topic because I think the simple factory will give you a good intuition for understanding the proper factory patterns.
Many online resources portray the simple factory as the factory method pattern, which is wrong.
You can read more about the terminology on Wikipedia.
What is a Simple Factory?
The simple factory is essentially a class that, based on a given input, returns objects of different types.
In contrast to the factory method or the abstract factory, the simple factory is made up of just one concrete factory. This concrete factory doesn’t inherit from another abstraction layer.
Just to reiterate, the simple factory is not considered a genuine pattern. That being said, use this concept as a stepping stone to understanding the factory method and the abstract factory.
To illustrate this pattern, let’s consider we’re a company that builds computers. For the moment, we’re only producing laptops.
In the class diagram below, the concept of laptop is shown via the Laptop
interface. We have two types of laptops which are represented by the NormalLaptop
and GamingLaptop
concrete classes that implement the Laptop
interface.
The client code is using the LaptopFactory
concrete factory class to create the objects it needs via the createLaptop()
method.
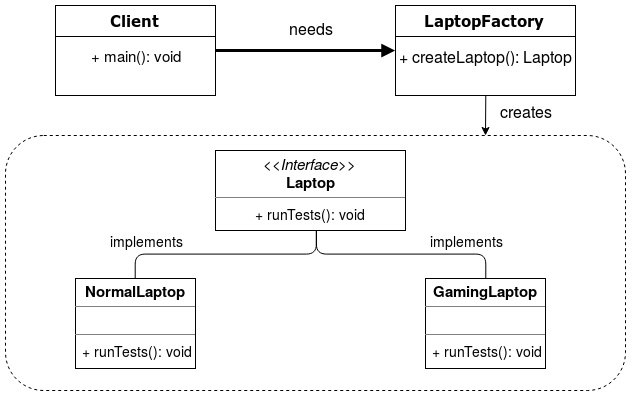
Simple Factory Class Diagram
Pros
- the same advantages mentioned for the factory method pattern such as encapsulating object creation apply.
Cons
- if a new type of Laptop is added to the system, then we’d also need to change the logic, i.e.
switch
statement, in the createLaptop()
method below. This can be avoided with the factory method pattern.
How Can You Implement the Simple Factory?
As usual, let’s implement in Java the design from the class diagram we saw in the previous section.
The Laptop
interface would look something like this:
The classes implementing the Laptop
interface are as follows:
Next, the object creation is done in LaptopFactory
based on information provided by the client code.
Lastly, the client code receives an object depending on the requested type:
The output would then be:
Summary
In conclusion, the two ideas you have to bear in mind are:
-
The simple factory is not a real pattern.
-
The simple factory is just the concrete factory, without the extra factory class abstraction found in either the factory method or abstract factory.