This blog post will help you understand how to use the strategy design pattern.
This post is part of the Design Patterns in Java Series. Check out the full list of covered patterns there.
Why Should You Use the Strategy Design Pattern?
The strategy design pattern is a behavioural pattern used when we need to dynamically select between different behaviours at runtime.
Essentially, the client code can select which behaviour should be used, without interfering with the code of the behaviours themselves.
Example use cases for using the strategy pattern would be choosing between different sorting algorithms depending on your input data, saving files in different formats, or selecting between different data validation methods.
What is the Strategy Pattern?
Here’s the definition from the Head First Design Patterns book:
The Strategy Pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. Strategy lets the algorithm vary independently from clients that use them.
There are three main participants in the strategy pattern:
-
Strategy – declares an interface that all supported strategies/algorithms must implement. A Context will use this interface to select a concrete strategy at runtime.
-
Concrete strategy – implements an algorithm defined by the Strategy interface.
-
Context – client code that keeps a reference to a Concrete strategy object, thus selecting at runtime which algorithm should be used.
Next, let’s take a look at the strategy pattern class diagram.
Just to mix it up a bit, let’s say one has the goal of losing weight. One can achieve this by weight lifting or cardio. Each of these is a behaviour/strategy to achieve the goal.
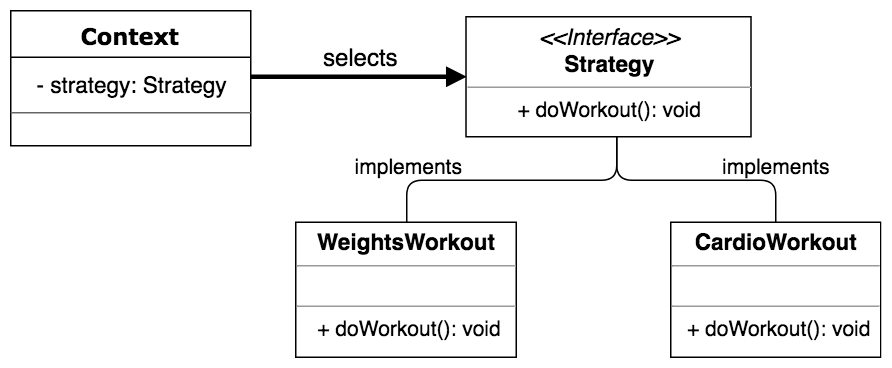
Strategy Pattern Class Diagram
The Context
class must choose at runtime one of the concrete strategies implementing the Strategy
interface, in this case WeightsWorkout
or CardioWorkout
.
Pros
- select algorithms at runtime – as we’re relying on composition instead of inheritance, the client code has more flexibility in terms of having the ability to select which strategy to select depending on different conditions.
- separation of concerns – the different algorithms are separated and the client code is not aware of how a behaviour is implemented.
Cons
- client code must know which strategy to choose.
- increased code complexity due to extra classes.
How Can You Implement the Strategy Pattern?
Let’s implement in Java the design from the class diagram in the previous section.
Strategy Pattern Java Code Example
The Strategy
interface is simple:
The two concrete strategies implementing the Strategy
interface are:
The Context
selecting the strategy would look like so:
The code to run the demo is:
The final output is then:
Summary
In conclusion, we have to remember that:
-
Strategy pattern defines a family of behaviours.
-
Strategy pattern makes it easy to switch between behaviours at runtime.