In this blog post, we’ll look into the factory method design pattern.
This post is part of the Design Patterns in Java Series. Check out the full list of covered patterns there.
Why Should You Use the Factory Design Pattern?
In object-oriented programming, we use objects. Groundbreaking stuff, am I right?
These objects need to be created somehow.
The factory pattern aims to control this creation in an isolated, restricted manner.
Therefore, if you need to create an object, you should ask the factory for one, instead of dealing with the instantiation yourself.
If you think about it, it makes sense to delegate this object creation to someone else (i.e. the factory), especially if this creation logic is complicated.
Thus, the factory encapsulates this fancy business logic and is responsible for creating new objects.
The Java example later in this post will make things clearer.
Factory Pattern Flavours
First of all, let’s establish that there are two definite factory patterns. In addition, there’s also what I like to call a pseudopattern that I want to touch upon in this section.
As one of the main goals of this blog is to make information easier to digest, I’ve decided to treat each of these related patterns within their own blog post.
That way, everyone’s happy.
Firstly, we have the simple factory pseudopattern, and I use the prefix pseudo because it’s actually not considered a pattern in the Gang of Four book. This is basically a class that, based on a given input, returns objects of different types.
I’ve written a small blog post on the simple factory here. Looking at the diagram there will help you develop better intuition about what we’re trying to learn here.
Also, the code example in this blog post builds on the one there, but don’t worry if you decide to skip that post, the code here is self-contained.
Secondly, there’s the factory method pattern. This is what this post is about.
Thirdly, we also have the abstract factory pattern. Briefly, this groups together multiple factories to make a “superfactory”. I’ve covered this in the abstract factory pattern blog post.
I recommend first understanding the current article, since the abstract factory pattern builds on the factory method.
What is the Factory Method?
The factory pattern is a creational pattern that decouples client code from object creation.
It has two purposes:
-
Creates objects.
-
Decouples clients from the creation of objects.
Here’s the definition from the Head First Design Patterns book:
Defines an interface for creating an object, but lets subclasses decide which class to instantiate.
Factory Method lets a class defer instantiation to subclasses.
As we said in the façade pattern post, an interface in this context refers to the concept of abstraction, of hiding away details.
Basically, the definition says that we trust the factory to create the object for us. In addition, the factory also decides the particular object to be created.
To illustrate this concept, let’s consider we’re running a computer manufacturing business. For the moment, we’re only focusing on laptops.
The Laptop
interface represents the laptops. This interface is implemented by the two available types of laptops pictured as the NormalLaptop
and GamingLaptop
concrete classes.
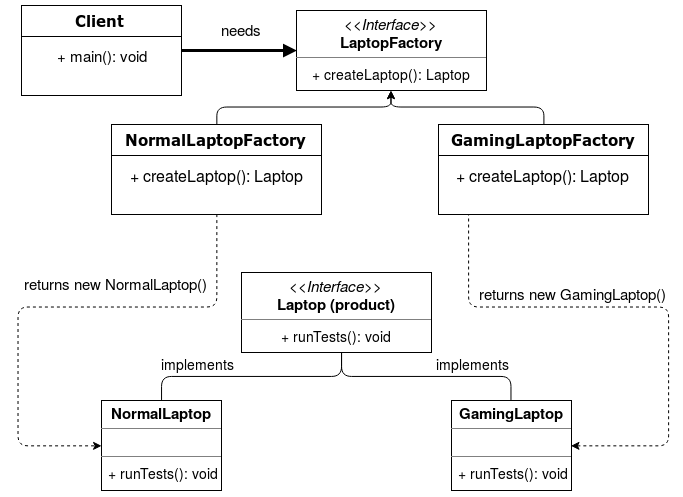
Factory Method Class Diagram
As per the definition of the factory method, we’ll need an interface for creating our objects. This is the role of the LaptopFactory
interface.
The next step is to create subclasses which will decide which of the two types of laptops will be instantiated. These subclasses are NormalLaptopFactory
and GamingLaptopFactory
, both implementing the LaptopFactory
interface.
The createLaptop()
methods of the concrete factories NormalLaptopFactory
and GamingLaptopFactory
decide which specific laptop class to instantiate.
Therefore, createLaptop()
is the factory method.
The Dependency Inversion Principle and the Factory
What we’re actually doing via the factory pattern is applying the Dependency Inversion Principle (DIP).
From the Agile Software Development, Principles, Patterns, and Practices book, the principle states:
- High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
In our case, an abstraction is the Laptop
interface. The client code is independent of the concrete classes that implement this interface, i.e. NormalLaptop
and GamingLaptop
.
As a bonus for you, the Professional Java EE Design Patterns book also states that we usually only need one instance of the factory.
Therefore, the factory pattern is often implemented as a singleton or as a static class.
Pros
- encapsulates object creation.
- decouples client code from object creation.
- isolates object creation logic, thus changes are made in only one place.
Cons
- added complexity – as with all patterns, overuse can hinder understanding of the system.
How Can You Implement the Factory Method Pattern?
Let’s implement in Java the design from the class diagram we saw previously.
Factory Method Pattern Java Code Example
The Laptop
interface would look something like this:
The classes implementing the Laptop
interface are as follows:
Next up is the LaptopFactory
interface.
The concrete factories that implement the createLaptop()
factory method are:
Lastly, the client code can be:
The output is then:
Summary
In conclusion, the three main ideas you have to remember are:
-
The factory pattern encapsulates object creation.
-
The factory pattern decouples client code from object creation.
-
The factory pattern gives your object creation code more flexibility and testability.